Not the first time i ran into problems with SVGs preserveAspectRatio attribute, but i can’t remember a case where Firefox handled this different from Chrome. However, lets have look at the this weird behavoir.
The goal was to build a very flexible lines-background-element with CSS-Grid:
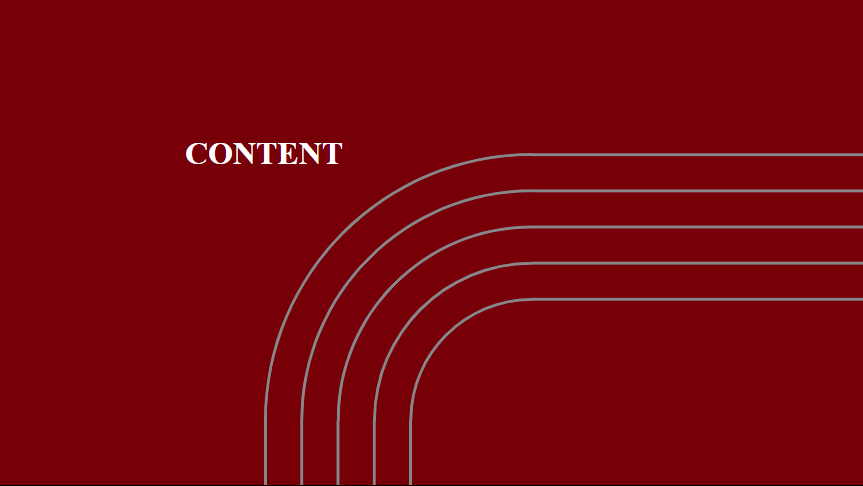
While Chrome renders everything perfectly fine, Firefox produced this:
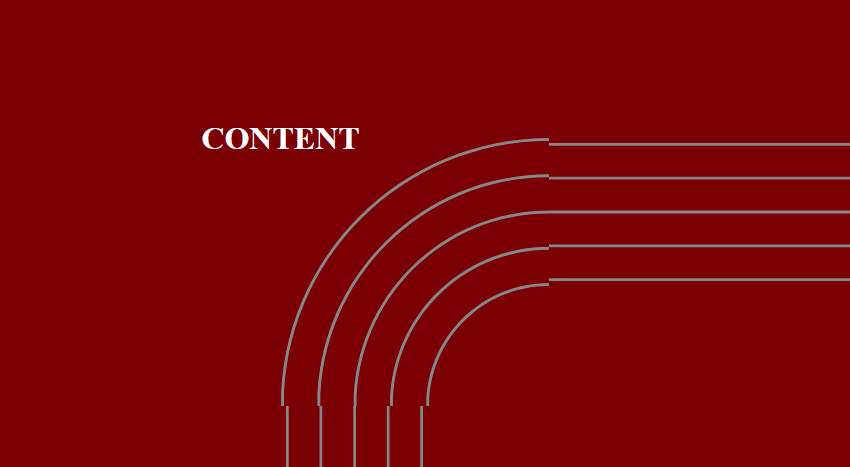
The Code:
HTML
<div class="line-bg line-bg--middle-center-to-bottom-right"> <div class="line-bg__item line-bg__item--curve-tl"></div> <div class="line-bg__item line-bg__item--flex-h"></div> <div class="line-bg__item line-bg__item--flex-v"></div> </div>
CSS
.line-bg{ --line-edge-group-size: 268px; --line-group-size: calc(var(--line-edge-group-size) * 0.5522); position:absolute; left:50%; top:50%; right:0; bottom:0; display:grid; grid-template-columns: var(--line-edge-group-size) auto; grid-template-rows: var(--line-edge-group-size) auto; } .line-bg__item--flex-v{ background-image: url('line_bg_v.svg'); background-repeat: repeat-y; background-position:0 0; background-size: var(--line-group-size) auto; }
SVG (the *wrong* code)
<?xml version="1.0" encoding="utf-8"?> <svg viewBox="0 0 690 25" xmlns="http://www.w3.org/2000/svg"> <g transform="matrix(1, 0, 0, 0.961538, 0, 0)"> <path d="M676.115,-0L676.115,26L690.031,26L690.031,-0L676.115,-0ZM507.101,-0L507.101,26L521.018,26L521.018,-0L507.101,-0ZM338.083,-0L338.083,26L352.004,26L352.004,-0L338.083,-0ZM169.069,-0L169.069,26L182.986,26L182.986,-0L169.069,-0ZM13.916,-0L0,-0L0,26L13.916,26L13.916,-0Z" style="fill:#878787;fill-rule:nonzero;"/> </g> </svg>
So after testing around, with the background-size, -position, etc. the conclusion was that the SVG itself must be the problem. And it kinda was, but i’im pretty sure its a Firefox Bug with SVG + background-size. As you can see in the code, i was not trying to change the aspect-ratio in any way, i just set a fixed with + auto height for the background and let em repeat verticaly, so the preserveAspectRatio shouldn’t needed here.
The Workaround
<?xml version="1.0" encoding="utf-8"?> <svg viewBox="0 0 690 25" xmlns="http://www.w3.org/2000/svg" preserveAspectRatio="none"> <g transform="matrix(1, 0, 0, 0.961538, 0, 0)"> <path d="M676.115,-0L676.115,26L690.031,26L690.031,-0L676.115,-0ZM507.101,-0L507.101,26L521.018,26L521.018,-0L507.101,-0ZM338.083,-0L338.083,26L352.004,26L352.004,-0L338.083,-0ZM169.069,-0L169.069,26L182.986,26L182.986,-0L169.069,-0ZM13.916,-0L0,-0L0,26L13.916,26L13.916,-0Z" style="fill:#878787;fill-rule:nonzero;"/> </g> </svg>
Set preserveAspectRatio=“none“ on the SVG wrapper. Done.